The CANVAS element is one of the cool additions to Html 5. You may have seen numerous web applications developed using Flash or Silverlight which support online coloring or painting on the web pages. No more Flash or Silverlight is needed for this kind of creative work. The CANVAS element in a very flexible element that allows you to use JavaScript to be really creative and make something cool on the web.
CANVAS element is introduced in HTML 5 and is used to create graphics on the web pages. CANVAS tag only provides the canvas to draw and does not support drawing by itself. You must use JavaScript or other scripting technologies to draw on the canvas. This is like the real canvas on real painting. It just provides a place to paint or draw. You still need other painting tools to paint on the canvas.
Here is the syntax for using CANVAS tag in HTML 5:
The above syntax shows the tag specific attributes of the CANVAS element. However, this tag supports several other Global attributes which are common for other HTML 5 elements, including:
In addition, CANVAS element supports a wide range of standard events like other HTML elements. JavaScript or other scripting languages can be used to track the events and take appropriate action based on the events.
You can draw graphics on the canvas or edit images using JavaScript or other scripting languages. Here is an example:
The text content within the <canvas></canvas> tells the browser to display that warning message to indicate that the browser does understand/support the tag.
The above html defines a canvas tag which is ready to draw on it. Now, you need to use JavaScript to get access to the canvas object and draw on it. Here is an example of drawing a rectangle on the given canvas using JavaScript.
The above JavaScript will draw a rectangle in the canvas.
If you are looking for the source code for a full sample of drawing rectangles see the below source code for a full html page. Copy the below code in to notepad and save it as an html file. Then open the html file in a browser to see the cool rectangles drawn on the canvas.
The above html will display the rectangles as shown here:
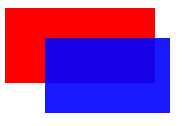
You can experiment more with Javascript on canvas, draw a lot more shapes and even do some basic animations. There are several games developed using HTML 5 and Javascript. AngryBirds has an HTML 5 version, which is a very popular game among kids.
In the upcoming chapters of this series of HTML 5 tutorials, I will cover more HTML 5 samples and mini projects.
What is the purpose of the CANVAS element in HTML5?
CANVAS element is introduced in HTML 5 and is used to create graphics on the web pages. CANVAS tag only provides the canvas to draw and does not support drawing by itself. You must use JavaScript or other scripting technologies to draw on the canvas. This is like the real canvas on real painting. It just provides a place to paint or draw. You still need other painting tools to paint on the canvas.
Syntax of CANVAS element in HTML 5
Here is the syntax for using CANVAS tag in HTML 5:
<CANVAS WIDTH=100px HEIGHT=200px> </CANVAS >
The above syntax shows the tag specific attributes of the CANVAS element. However, this tag supports several other Global attributes which are common for other HTML 5 elements, including:
accesskey class contenteditable contextmenu dir draggable hidden id lang spellcheck style tabindex title
In addition, CANVAS element supports a wide range of standard events like other HTML elements. JavaScript or other scripting languages can be used to track the events and take appropriate action based on the events.
How to draw on canvas element using JavaScript
You can draw graphics on the canvas or edit images using JavaScript or other scripting languages. Here is an example:
<canvas id="myDrawing" width="250" height="250"> Your browser doesn't support canvas. </canvas>
The text content within the <canvas></canvas> tells the browser to display that warning message to indicate that the browser does understand/support the tag.
The above html defines a canvas tag which is ready to draw on it. Now, you need to use JavaScript to get access to the canvas object and draw on it. Here is an example of drawing a rectangle on the given canvas using JavaScript.
// Get the element from the html DOM var drawingCanvas = document.getElementById('myDrawing'); // Check if the element is in the DOM and the browser supports canvas element if (drawingCanvas && drawingCanvas.getContext) { // Initaliase a 2-dimensional drawing context var context = drawingCanvas.getContext('2d'); // Draw a rectangle context.fillStyle = "rgb(300,0,0)"; context.fillRect(0, 0, 150, 75); } </script>
The above JavaScript will draw a rectangle in the canvas.
If you are looking for the source code for a full sample of drawing rectangles see the below source code for a full html page. Copy the below code in to notepad and save it as an html file. Then open the html file in a browser to see the cool rectangles drawn on the canvas.
<!DOCTYPE html /> <html xmlns="http://www.w3.org/1999/xhtml" > <head> <title>Canvas HTML 5 element example</title> </head> <body> <canvas id="myDrawing" width="250" height="250"> Your browser doesn't support canvas. </canvas> <script language="javascript"> // Get the element from the html DOM var drawingCanvas = document.getElementById('myDrawing'); // Check if the element is in the DOM and the browser supports canvas element if (drawingCanvas && drawingCanvas.getContext) { // Initaliase a 2-dimensional drawing context var context = drawingCanvas.getContext('2d'); // Draw a rectangle context.fillStyle = "rgb(255,0,0)"; context.fillRect(0, 0, 150, 75); // Draw another rectangle with some transparency context.fillStyle = "rgba(0, 0, 255, 0.9)"; context.fillRect(40, 30, 125, 75); // Draw another rectangle border without filling. context.fillStyle = "rgb(0,0,150)"; context.strokeRect(20, 20, 50, 100); } </script> </body> </html>
The above html will display the rectangles as shown here:
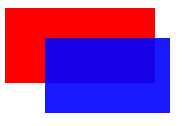
You can experiment more with Javascript on canvas, draw a lot more shapes and even do some basic animations. There are several games developed using HTML 5 and Javascript. AngryBirds has an HTML 5 version, which is a very popular game among kids.
In the upcoming chapters of this series of HTML 5 tutorials, I will cover more HTML 5 samples and mini projects.